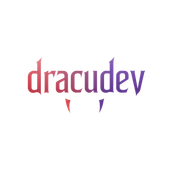
Javier Andreu
Front-End Developer
Barcelona, Spain
Front-End developer focused on creating dynamic web applications with React, TypeScript, and Tailwind, taking care of every detail to ensure clean, scalable, and efficient code.
I also have a background in digital marketing and UX, which underscores the importance of developing applications that not only work effectively but also provide an intuitive and optimized user experience.
interface DeveloperConfig {
techStack: {
[category: string]: string[];
};
softSkills: string[];
openToWork: boolean;
}
const javierAndreu: DeveloperConfig = {
techStack: {
frontendFrameworks: [
"React.js",
"Next.js",
"Astro.js",
],
styling: [
"Tailwind CSS",
"CSS Modules",
"Styled-Components",
"SASS/SCSS",
"Bootstrap",
],
stateManagement: [
"Redux Toolkit",
"Zustand",
"Context API",
],
backendTechnologies: [
"Node.js",
"Express.js",
"Prisma",
"Supabase",
"MySQL"
],
testingTools: [
"Jest",
"React Testing Library",
"Storybook"
],
devopsTools: [
"Git",
"GitHub Actions",
"Vercel"
],
performanceTools: [
"Lighthouse",
"Chrome DevTools",
"React Profiler"
]
},
softSkills: [
"Agile Methodology",
"Team Collaboration",
"Problem-Solving",
"Quick Learning",
"Communication",
"Adaptability"
],
openToWork: true
};
// Contact function
type ContactType = 'github' | 'linkedIn' | 'email';
const contact = (type: ContactType): string => {
switch (type) {
case 'github':
return 'https://github.com/dracudev';
case 'linkedIn':
return 'https://www.linkedin.com/in/javier-andreu-peralta/';
case 'email':
return 'andreujavier99@gmail.com';
default:
return 'Invalid contact type';
}
};
// Career Objectives
const careerObjective = `Seeking an opportunity to leverage my technical skills
and passion for creating innovative web solutions in a dynamic,
growth-oriented frontend development role. Committed to continuous
learning and delivering high-quality, user-centric applications.`;
El Gavilán - Ecommerce
E-commerce platform for a traditional hat store using Next.js, React, TypeScript, and Tailwind CSS. Implemented a secure checkout with Stripe, a user authentication with Supabase, and an admin panel.
Bel'Pom - Corporate Website
Corporate website for potato factory Bel'pom using Astro.js, TypeScript, and Tailwind CSS. Implementation of a modern, responsive interface to present the company, its products, and service information.
Regame - Trading Platform
Video game trading platform with a Node.js API and MySQL database, featuring a React front end with a trade map, calendar with events, and charts to track exchanges and game popularity.
Star Wars Guide - Consumed API
Star Wars visual guide using the SWAPI API with React, TypeScript, and Tailwind CSS. Implemented pagination, user authentication, protected routes, and a detailed view of characters, films, and starships.
Budget App - Projects Pricing
Budget app to calculate project pricing using React, JavaScript, and Bootstrap. Implemented a form to add projects, calculate prices, and display a list of projects with their total cost.
:root {
--current-role: "Freelance Front-End Developer";
--current-period: "Jan 2025 - Present";
--current-location: "Barcelona, Catalonia, Spain · Remote";
}
/* Freelance Front-End Developer */
.professional-experience--freelance {
work-period: "Jan 2025 - Present";
employment-type: autonomous;
projects: [
/* Project: El Gavilán */
{
client: "El Gavilán",
project-type: "E-commerce Platform",
technologies: [
"Next.js",
"React",
"TypeScript",
"Tailwind CSS"
],
key-features: [
"Secure Stripe Payment Integration",
"Supabase User Authentication",
"Admin Product Management Panel"
]
},
/* Project: Bel'pom */
{
client: "Bel'pom",
project-type: "Corporate Website",
technologies: [
"Astro.js",
"React",
"TypeScript",
"Tailwind CSS"
],
key-features: [
"Modern Responsive Interface",
"Company Information Showcase",
"Services Presentation"
]
}
];
skills: [
"React.js",
"Node.js",
"Tailwind CSS",
"Web Development",
"TypeScript",
"Next.js",
"Stripe",
"Supabase",
"Astro.js"
];
}
/* Sales Assistant Experience */
.professional-experience--sales-assistant {
company: "Sombrerería El Gavilán";
work-period: "Sept 2023 - Aug 2024";
employment-type: full-time;
location: "Orihuela, Valencia, Spain";
responsibilities: [
"Traditional Business Digitalization",
"SEO/SEM Strategy Implementation",
"Visual Merchandising Support",
"Personalized Customer Service"
];
skills: [
"Teamwork",
"Social Media",
"SEO Optimization",
"Web Development",
"Visual Merchandising",
"Community Management",
"Digital Marketing",
"SEM",
"Customer Service"
];
}
/* Junior Marketing Role */
.professional-experience--marketing {
company: "TD SYNNEX";
work-period: "May 2022 - Apr 2023";
employment-type: full-time;
location: "Barcelona, Catalonia, Spain · Hybrid";
responsibilities: [
"Customer Relationship Management (CRM - SAP)",
"Digital Campaign Content Layout",
"Market Trend Analysis",
"International Digital Marketing Projects"
];
skills: [
"Digital Marketing",
"Market Research",
"CRM Management",
"SAP CRM",
"Teamwork",
"Marketing",
"Customer Orientation",
"Adobe Photoshop",
"Microsoft Office"
];
}
/* Metadata */
@meta-info {
last-updated: "2024-03-26";
resume-version: "1.0.0";
professional-status: "Active";
}
{
"education": [
{
"institution": "IT Academy de Barcelona Activa",
"degree": "Web Application Development Bootcamp",
"period": "Oct 2024 - Jun 2025",
"@skills": [
"Web Development",
"Git",
"JavaScript",
"TypeScript",
"React.js",
"Bootstrap",
"Tailwind CSS",
"MySQL",
"MongoDB",
"Node.js",
"Express.js"
]
},
{
"institution": "Autonomous University of Barcelona",
"degree": "Master in Strategic Planning in Advertising and PR",
"period": "Sep 2021 - Jul 2022",
"@skills": [
"Strategic Communication",
"Market Research",
"Digital Marketing",
"SEO",
"E-commerce Strategies",
"Brand Planning"
]
},
{
"institution": "Complutense University of Madrid",
"degree": "Bachelor in Public Relations and Applied Communication",
"period": "2017 - 2021",
"@skills": [
"Communication Theory",
"Digital Media",
"Public Relations",
"Advertising Principles",
"Media Management"
]
}
],
"certifications": [
{
"name": "Responsive Web Design",
"issuer": "freeCodeCamp",
"date": "May 2024",
"@skills": [
"HTML5",
"CSS",
"Web Accessibility"
],
"credential": "dracudev-rwd"
},
{
"name": "Full Stack Web Programming",
"issuer": "Adecco",
"date": "Dec 2023",
"@skills": [
"Full Stack Development",
"JavaScript Ecosystem",
"Backend Technologies"
]
}
],
}